介绍
一个支持多种存储的文件列表程序
官方文档
Home | AList文档 (nn.ci)
运行截图
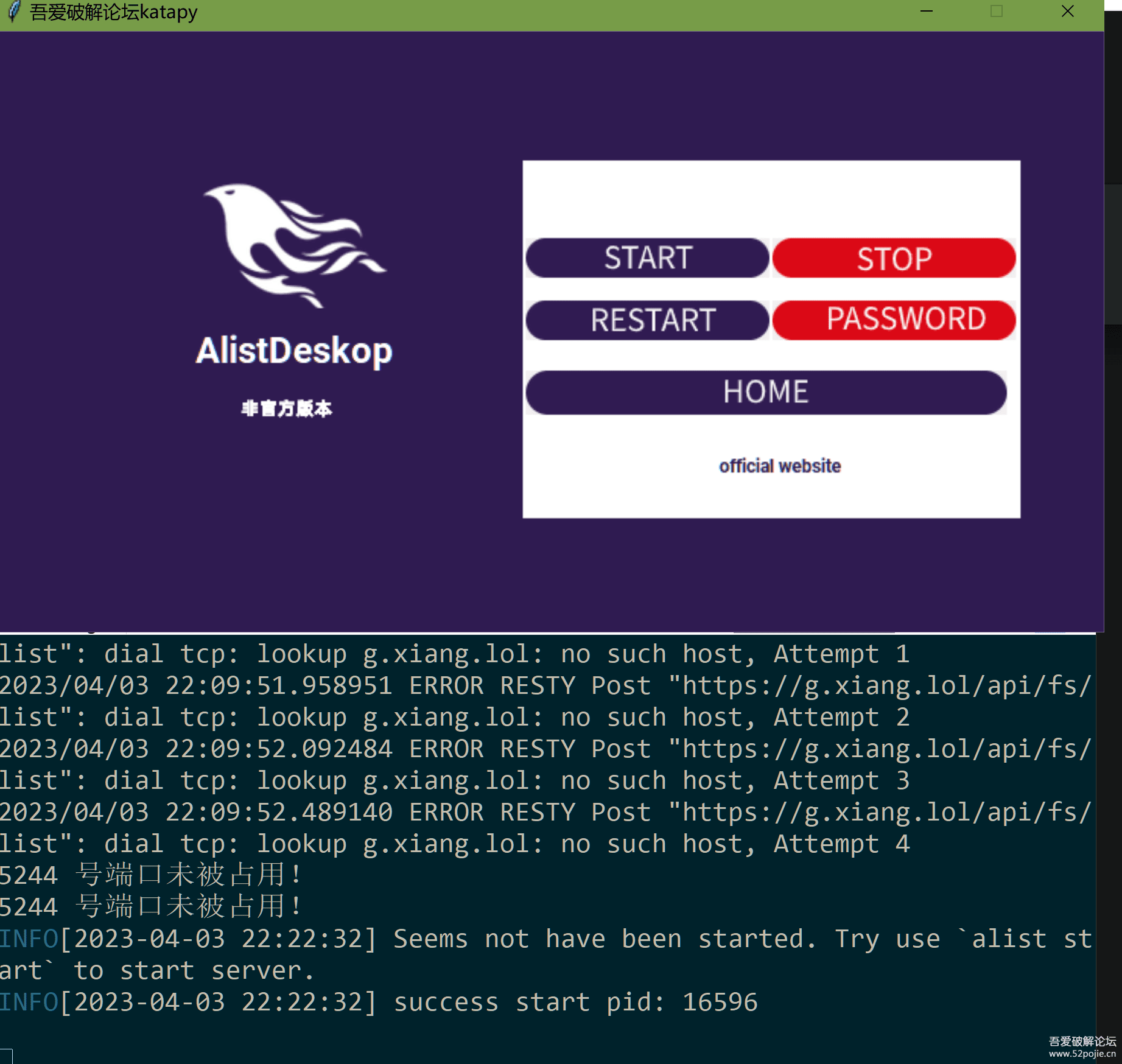
源码
# -*-coding:utf-8-*-
import os
import threading
from pathlib import Path
import subprocess
import asyncio
from asyncio.windows_events import ProactorEventLoop
from tkinter import Tk, Canvas, Entry, Text, Button, PhotoImage
OUTPUT_PATH = Path(__file__).parent
ASSETS_PATH = OUTPUT_PATH / Path(r"C:\Users\ins\Desktop\adsf\build\assets\frame0")
def relative_to_assets(path: str) -> Path:
return ASSETS_PATH / Path(path)
# 异步执行函数
def btn_clicked():
async def _btn_clicked():
proc = await asyncio.create_subprocess_exec(
'./alist-windows-amd64/alist.exe',
'server',
stdout=asyncio.subprocess.PIPE)
stdout, stderr = await proc.communicate()
print(stdout.decode())
asyncio.run(_btn_clicked())
def on_btn_clicked():
threading.Thread(target=btn_clicked).start()
# 停止服务
async def btn_clicked_stop(port):
"""
利用命令行方式杀掉指定端口号的进程,实现释放端口的效果。
"""
loop = asyncio.get_running_loop()
if os.name == 'nt':
# Windows系统下的命令
command = f"netstat -ano | findstr {port} | findstr LISTENING"
procommand = subprocess.Popen(command, shell=True, stdout=subprocess.PIPE, stderr=subprocess.PIPE)
out, err = await loop.run_in_executor(None, procommand.communicate)
pid = str(out).split()[-1].strip()
if pid.isnumeric():
cmd = f"taskkill /f /pid {pid}"
await loop.run_in_executor(None, os.system, cmd)
print(f'成功关闭 {port} 号端口!')
else:
print(f'{port} 号端口未被占用!')
else:
# Linux/Mac系统下的命令
command = f"lsof -i :{port} -sTCP:LISTEN -t"
procommand = subprocess.Popen(command, shell=True, stdout=subprocess.PIPE, stderr=subprocess.PIPE)
out, err = await loop.run_in_executor(None, procommand.communicate)
pid = str(out.strip(), encoding='utf-8')
if pid.isnumeric():
cmd = f"kill -9 {pid}"
await loop.run_in_executor(None, os.system, cmd)
print(f'成功关闭 {port} 号端口!')
else:
print(f'{port} 号端口未被占用!')
def on_btn_clicked_stop():
loop = ProactorEventLoop()
asyncio.set_event_loop(loop)
loop.run_until_complete(btn_clicked_stop(5244))
loop.close()
# 重启服务
async def btn_clicked_res():
proc = await asyncio.create_subprocess_exec('./alist-windows-amd64/alist.exe', 'restart', stdout=asyncio.subprocess.PIPE)
stdout, stderr = await proc.communicate()
print(stdout.decode())
def on_btn_clicked_res():
loop = ProactorEventLoop()
asyncio.set_event_loop(loop)
loop.run_until_complete(btn_clicked_res())
loop.close()
import webbrowser
async def open_google():
"""
打开 首页。
"""
url = 'http://127.0.0.1:5244'
webbrowser.open(url)
def on_btn_clicked_home():
loop = ProactorEventLoop()
asyncio.set_event_loop(loop)
loop.run_until_complete(open_google())
loop.close()
async def open_event():
"""
打开 首页。
"""
url = 'https://alist.nn.ci/zh/guide/'
webbrowser.open(url)
def on_btn_clicked_guide(event):
loop = ProactorEventLoop()
asyncio.set_event_loop(loop)
loop.run_until_complete(open_event())
loop.close()
window = Tk()
window.geometry("727x395")
window.configure(bg = "#FFFFFF")
canvas = Canvas(
window,
bg = "#FFFFFF",
height = 395,
width = 727,
bd = 0,
highlightthickness = 0,
relief = "ridge"
)
canvas.place(x = 0, y = 0)
canvas.create_rectangle(
0.0,
0.0,
727.0,
395.0,
fill="#2F1B51",
outline="")
canvas.create_rectangle(
345.0,
85.0,
672.0,
320.0,
fill="#FFFFFF",
outline="")
button_image_1 = PhotoImage(
file=relative_to_assets("button_1.png"))
button_1 = Button(
image=button_image_1,
borderwidth=0,
highlightthickness=0,
command = on_btn_clicked,
relief="flat"
)
button_1.place(
x=347.0,
y=136.0,
width=160.0,
height=26.0
)
button_image_2 = PhotoImage(
file=relative_to_assets("button_2.png"))
button_2 = Button(
image=button_image_2,
borderwidth=0,
highlightthickness=0,
command=on_btn_clicked_res,
relief="flat"
)
button_2.place(
x=347.0,
y=177.0,
width=160.0,
height=26.0
)
button_image_3 = PhotoImage(
file=relative_to_assets("button_3.png"))
button_3 = Button(
image=button_image_3,
borderwidth=0,
highlightthickness=0,
command=on_btn_clicked_home,
relief="flat"
)
button_3.place(
x=347.0,
y=223.0,
width=316.0,
height=29.0
)
button_image_4 = PhotoImage(
file=relative_to_assets("button_4.png"))
button_4 = Button(
image=button_image_4,
borderwidth=0,
highlightthickness=0,
command=on_btn_clicked_stop,
relief="flat"
)
button_4.place(
x=509.0,
y=136.0,
width=160.0,
height=26.0
)
button_image_5 = PhotoImage(
file=relative_to_assets("button_5.png"))
button_5 = Button(
image=button_image_5,
borderwidth=0,
highlightthickness=0,
command=on_btn_clicked_home,
relief="flat"
)
button_5.place(
x=509.0,
y=177.0,
width=160.0,
height=26.0
)
canvas.create_text(
413.0,
141.0,
anchor="nw",
text="start",
fill="#FFFFFF",
font=("Roboto Bold", 14 * -1)
)
canvas.create_text(
160.0,
241.0,
anchor="nw",
text="非官方版本",
fill="#FFFFFF",
font=("Roboto Bold", 12 * -1)
)
canvas.create_text(
587.0,
141.0,
anchor="nw",
text="stop",
fill="#FFFFFF",
font=("Roboto Bold", 14 * -1)
)
canvas.create_text(
573.0,
182.0,
anchor="nw",
text="password",
fill="#FFFFFF",
font=("Roboto Bold", 14 * -1)
)
canvas.create_text(
130.0,
195.0,
anchor="nw",
text="AlistDeskop",
fill="#FFFFFF",
font=("Roboto Bold", 24 * -1)
)
canvas.create_text(
408.0,
184.0,
anchor="nw",
text="restart",
fill="#FFFFFF",
font=("Roboto Bold", 14 * -1)
)
canvas.create_text(
451.0,
230.0,
anchor="ne",
text="open alist system",
fill="#2F1B51",
font=("Roboto Bold", 12 * -1)
)
canvas.create_text(
474.0,
279.0,
anchor="nw",
text="official website",
fill="#2F1B51",
font=("Roboto Bold", 12 * -1),
tags="official_website"
)
canvas.tag_bind("official_website", "<Button-1>", on_btn_clicked_guide)
image_image_1 = PhotoImage(
file=relative_to_assets("image_1.png"))
image_1 = canvas.create_image(
195.0,
141.0,
image=image_image_1
)
window.title("吾爱破解论坛katapy")
window.resizable(False, False)
window.mainloop()
来源:吾爱破解